If you’re building a website with MySQL and PHP, security should be one of your top priorities. Hackers are always looking for vulnerabilities, and an insecure database can put your entire site at risk. Additionally, If you are using WordPress, Understanding How to Secure MySQLi, Rename URLs, and Manage Redirection URLs in WordPress is crucial whether it’s for site migration or restructuring. Handling URL redirections properly is also key to keeping your visitors happy and your SEO intact.
In this guide, we’ll break things down into three essential topics: securing MySQLi connections, renaming URLs in WordPress, and managing redirections smoothly.
Securing MySQLi Connections
MySQLi (MySQL Improved) is a more secure and efficient way to connect PHP applications to a MySQL database. However, using it incorrectly can expose your site to serious threats like SQL injections and unauthorized data access.
1. Use Prepared Statements
One of the biggest mistakes developers make is using raw SQL queries with user input, which can lead to SQL injection attacks. The best way to prevent this is by using prepared statements.
Example:
<?php
$mysqli = new mysqli("localhost", "username", "password", "database");
if ($mysqli->connect_error) {
die("Connection failed: " . $mysqli->connect_error);
}
$stmt = $mysqli->prepare("SELECT * FROM users WHERE email = ?");
$stmt->bind_param("s", $email);
$email = "[email protected]";
$stmt->execute();
$result = $stmt->get_result();
while ($row = $result->fetch_assoc()) {
echo $row['name'];
}
$stmt->close();
?>
This ensures that user input is safely handled and prevents malicious SQL injection attempts.
2. Use SSL for Secure Connections
To protect data transmission between your server and the MySQL database, always use SSL encryption.
Example:
$mysqli = new mysqli('localhost', 'username', 'password', 'database', 3306, '/path/to/mysql/socket');
$mysqli->ssl_set(NULL, NULL, '/path/to/ca-cert.pem', NULL, NULL);
$mysqli->real_connect();
3. Use Strong Passwords and Limited Privileges
- Choose a strong password with a mix of uppercase, lowercase, numbers, and special characters.
- Avoid using the default “root” account for your database.
- Restrict database privileges—only grant users the permissions they absolutely need.
4. Keep MySQL and MySQLi Updated
Updates often include security patches, so make sure both your MySQL server and PHP’s MySQLi extension are always up to date.
Renaming URLs in WordPress
Changing your site’s URL in WordPress is common when moving to a new domain or switching from HTTP to HTTPS. WordPress stores URLs in various database tables, so you’ll need to update them properly.

1. Update the wp_options Table
WordPress keeps the primary site URL and home URL in the wp_options table. To update them, run this SQL command:
UPDATE wp_options
SET option_value = 'https://newdomain.com'
WHERE option_name = 'siteurl' OR option_name = 'home';
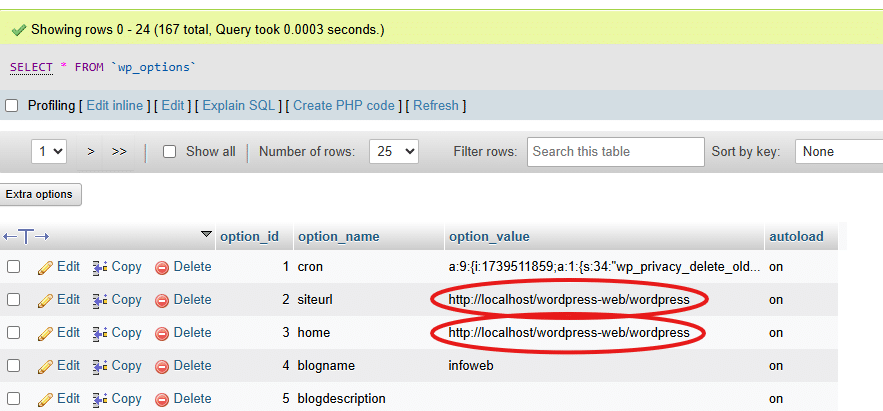
2. Update Internal Links in wp_posts Table
Your post content may contain old URLs that need updating. Use this query:
UPDATE wp_posts
SET post_content = REPLACE(post_content, 'https://olddomain.com', 'https://newdomain.com');
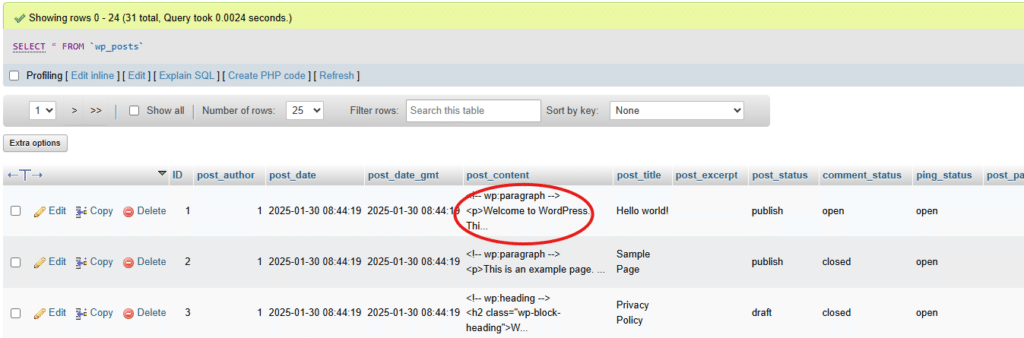
3. Update URLs in wp_postmeta Table
Some themes and plugins store URLs in wp_postmeta. Update them with this:
UPDATE wp_postmeta
SET meta_value = REPLACE(meta_value, 'https://olddomain.com', 'https://newdomain.com');
Managing Redirections in WordPress
When changing URLs, you need to set up proper redirections to ensure visitors and search engines don’t end up on broken links.
1. Use .htaccess for Redirection
If you’re changing your domain, modify your (.htaccess) file to redirect visitors:
RewriteEngine On
RewriteCond %{HTTP_HOST} ^olddomain\.com [NC]
RewriteRule ^(.*)$ https://newdomain.com/$1 [L,R=301]
This ensures that every page from the old domain redirects to the same page on the new domain.
2. Use a WordPress Plugin
If you’re not comfortable editing (.htaccess), install a plugin like Redirection to manage redirects through WordPress.
3. Redirect via PHP
For custom redirections, you can use PHP to redirect visitors:
<?php
header("Location: https://newdomain.com", true, 301);
exit();
?>
This permanently redirects users to the new URL.
Conclusion
Keeping your WordPress site secure and well-maintained involves more than just installing security plugins.
- Securing MySQLi: Always use prepared statements, SSL encryption, and strong passwords.
- Renaming URLs: Update the wp_options, wp_posts, and wp_postmeta tables when changing your domain.
- Managing Redirections: Use (.htaccess), plugins, or PHP scripts to redirect visitors smoothly.
By following these steps, you’ll ensure your website stays secure, functional, and SEO-friendly